The Logic Within: Demystifying Conditional Statements and Loops
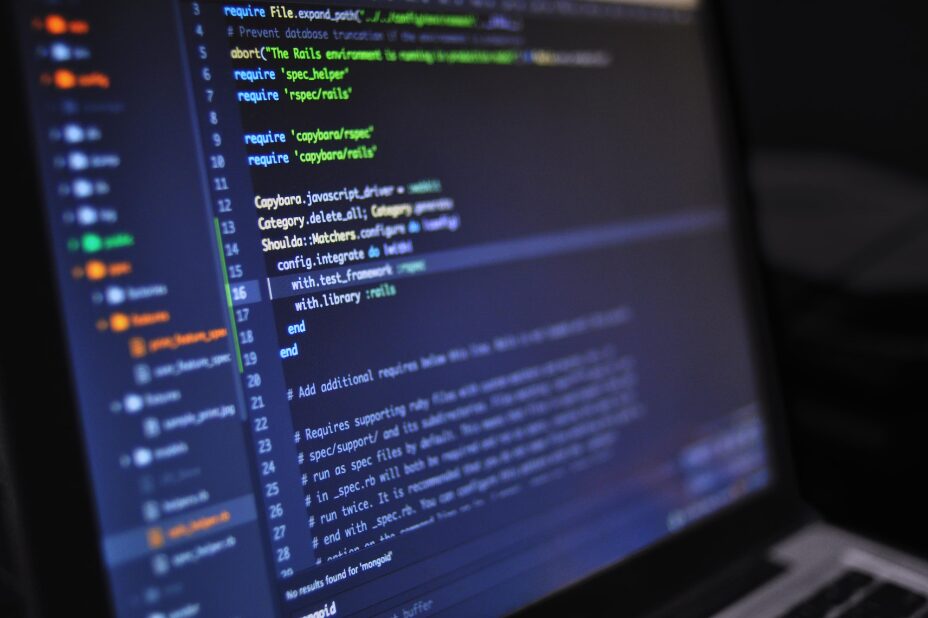
Build strong coding foundations by learning the coding building blocks. You can apply these concepts in almost any programming language.
Simple programs are run sequentially. Instructions are written line by line and the computer executes them exactly as written every time. However, for complex programs we have to make decisions based on various parameters. For example, a weather app shows different visuals based on the temperature or time of day. We looked at a few foundational programming concepts in a previous article. In this article, we’ll be taking a look at two more coding building blocks: conditional statements and loops.
Both conditionals and loops are essential in creating complex programs. They allow us to create branches in our programs or automate certain types of instruction. Almost every programming language you learn will have syntax for conditional statements and loops.
In this post, we will be exploring the following:
- If statement
- If-Else statement
- For loop
If Statement
Code will run sequentially unless specified otherwise. If there are any errors, the program might show an error or retry the instruction that caused the error. But even then, the code would still be considered “unconditional.” Errors are exceptional cases and are not part of the regular program flow. “Unconditional” code refers to code that is written in a sequential manner without any logic applied. Wouldn’t it be great if we could say “If this is true . . . do this thing first”? Well, it turns out, we can! Statements and logic like that are called conditionals, where we modify the flow of the program with some logic.
Consider a program in JavaScript where we want to alert a student if he or she passed a test:
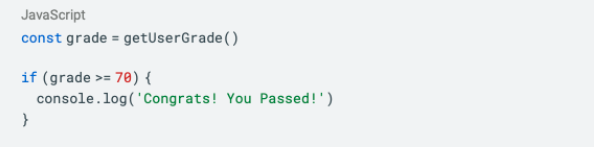
The if statement allows us to specify a “condition.” In JavaScript, an if statement is immediately followed by an open parenthesis (, a “conditional clause,” and a close parenthesis ). The conditional clause is any expression that can evaluate to either “true” or “false.”
In this case, either the grade is greater than or equal to 70, or it’s not. If it’s greater than or equal to 70, then the program will execute the content of the if block. If the grade is less than 70, then the program will not execute the content of the if block. Consider the following flowchart:
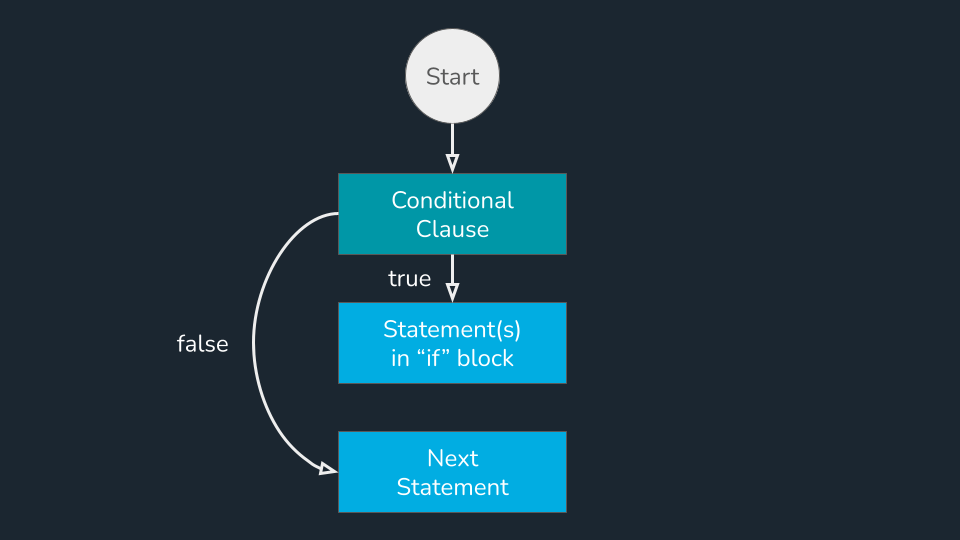
If-Else Statement
An if statement can be followed by an else statement. When an else follows an if, its content will be executed whenever the conditional clause of the if statement is not true. It should be noted that an if statement does not need to be followed by an else statement, as we have seen in the example above. But if we are to have an else statement, the syntax would look like this:
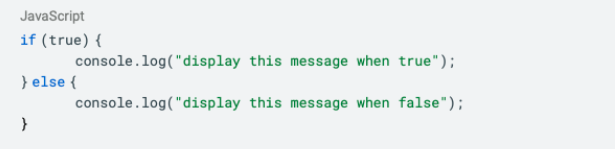
Consider the following flowchart to summarize how an if-else statement works:
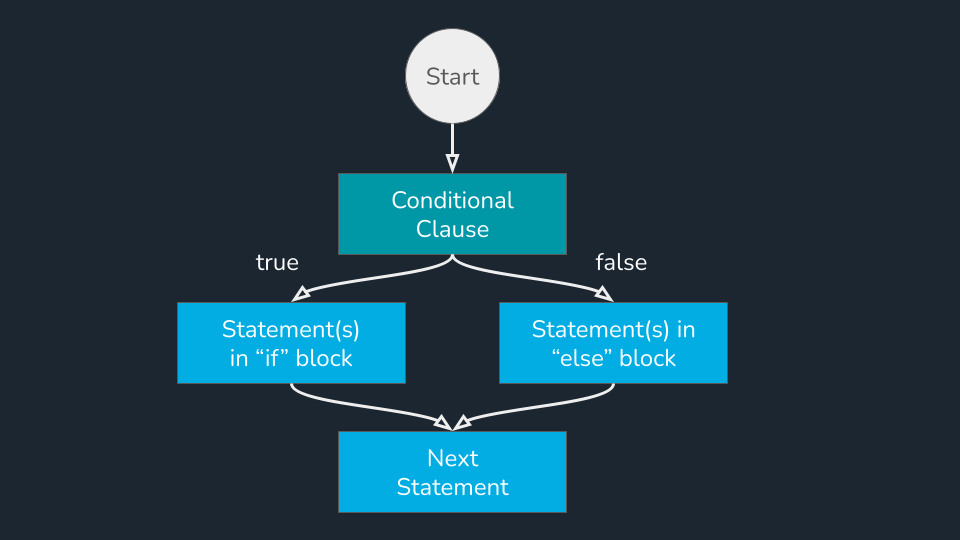
if-else statements are a good option to use when we have exactly two cases. In the example we’re working with, a student will either pass or fail. Another example of having exactly two cases would be determining if a number is even or odd.
For Loop
We can loop through the same block of code multiple times given some conditions. Loops are helpful if we want to execute the same statements over a number of iterations. Consider the following pseudocode:
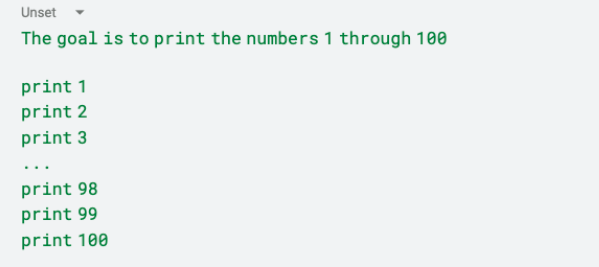
Notice how we would have to write a hundred console.log statements if we were to write out this pseudocode in JavaScript. But the pattern seems to be that we are executing a print statement a hundred times and just changing the value of what is printed. While this code is rather simplistic in that it is only one line of code that we want to repeat over and over, imagine if it was several lines of code that we wanted to repeat over and over? This could cause a maintenance nightmare of having to update repeated lines of code if we just want to make one little change! This is a good case to implement a loop that will save us from several copy-paste issues when developing and maintaining.
The for loop is a way to indicate that we want to go through a section of code multiple times. The for loop takes multiple parameters:
- An initializing statement that is run once before the content of the for loop.
- A conditional that is evaluated once before each run through the for loop and must be true in order for the content of the for loop to be executed.
- An update statement that is run once after each run through the for loop.
The syntax is as follows:
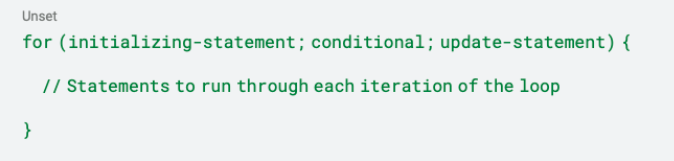
This kind of loop is very well suited in cases where we know in advance how many times we want to go through a specific set of instructions. Consider the following flowchart:
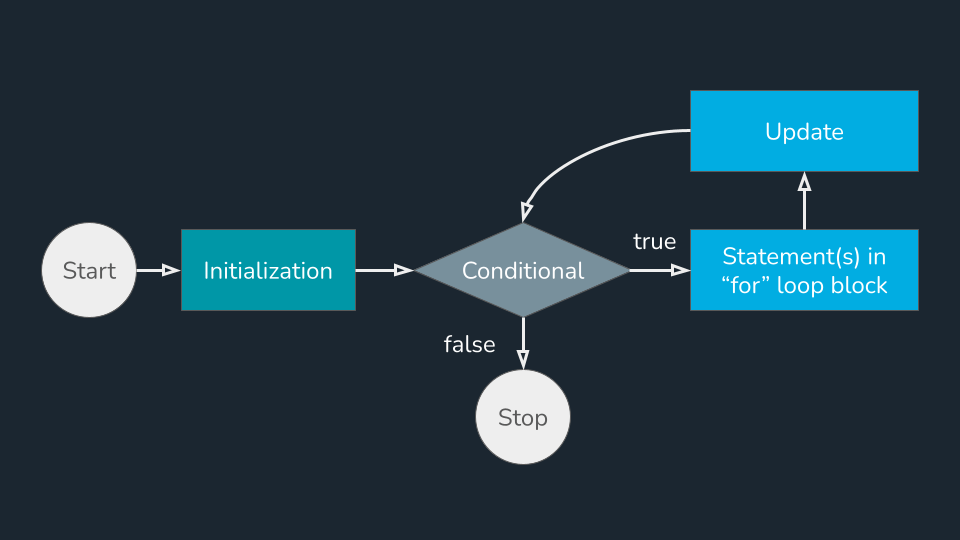
Let’s consider that we want to print a message to the console five times. We would write the following code:

Let’s break down what this for loop does:
- Define a variable named i and initialize it to 0.
- Establish that the code inside the for loop code block should run as long as i is less than 5.
- Establish that the value of i should be incremented by one every time the loop is executed.
We can revise our flowchart from before to reflect this example:
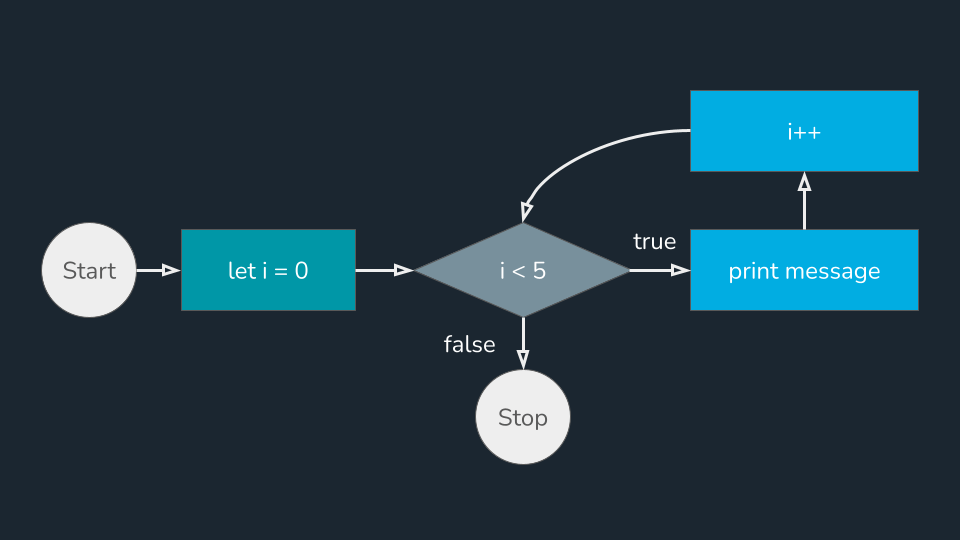
This will produce the following output:
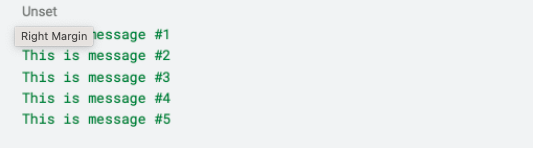
Conditional statements and loops will allow you to develop programs that can react to your user’s choices and automate or streamline various workflows. You can create delightful, interactive experiences for users by leveraging different control flow strategies.
Learn Software Engineering at Flatiron School
Our Software Engineering Bootcamp allows students to gain an education in the field in as little as 15 weeks. You can apply today or book a call with our Admissions team to learn more! And if you are interested in seeing the types of projects you can work on at Flatiron, attend our Final Project Showcase.
Disclaimer: The information in this blog is current as of March 29, 2024. Current policies, offerings, procedures, and programs may differ.