The Building Blocks of Code: Understanding Programming Variables, Data Types, and Operators
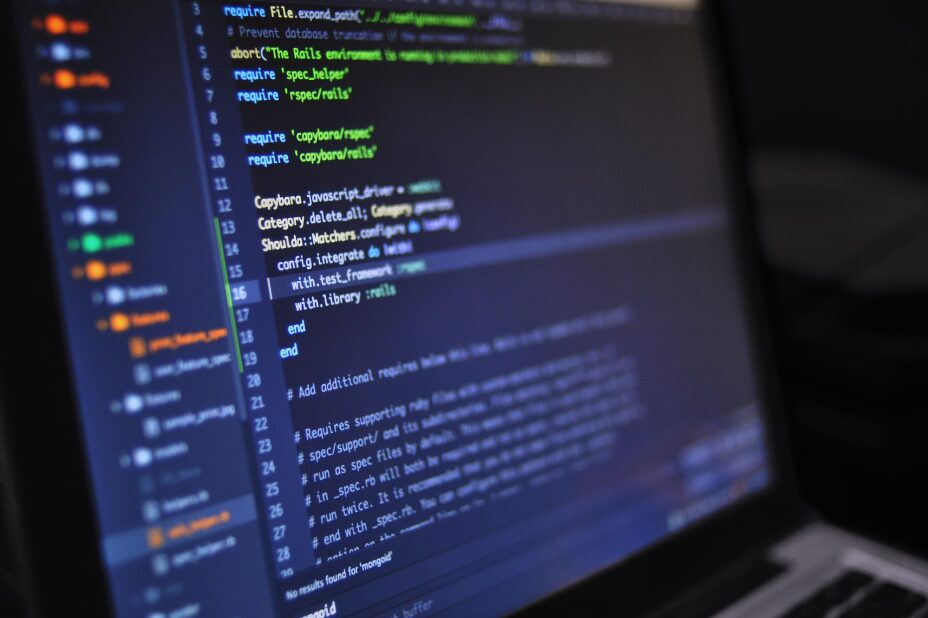
Learn some of the foundational building blocks of programming languages so you can start learning to code in any language.
Most software engineers end up using multiple programming languages throughout their careers. The great thing about programming languages is that certain core principles or concepts translate easily across languages. Once you learn and understand the building blocks of code, you’ll be able to pick up any technology on your own. Programming variables, data types, and operators are three of the most common building blocks across programming languages. In this post, we will be briefly exploring each of these fundamental concepts.
I’ll be using Python examples since concrete examples are more illustrative. But the concepts will easily translate to other languages.
Understanding Data Types in Programming
A computer does not understand information like humans do. For example, when we look at a text or string like “Hi! My name is Al!” or a number like “10” we can distinguish between them easily. We understand that strings and numbers have different properties and are used in various contexts.
The computer cannot distinguish between different types of information automatically. There are unique ways to represent values so that the computer can differentiate them. Each unique type of value is assigned a “data type” to classify or organize them, allowing us to know what contexts a certain value can be used.
We will look at the most common data types used in Python:
- str: Represents text or string values
- int: Represents integer number values (whole numbers like 1, 5)
- float: Represents floating-point numbers (decimal numbers like 1.556, 3.14)
- bool: Represents the idea of correctness, (i.e., whether something is true or false)
String Values
We use the values of the str data type to represent textual information to the computer. Quotation marks are usually used to denote text as “string” in programming languages.
Here are some examples in Python:
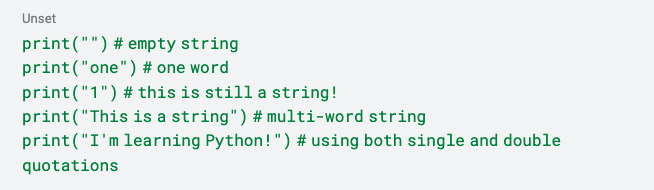
Here’s the output of the above code:
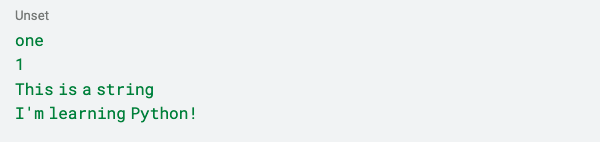
Notice a few important things:
- The text is displayed exactly as it is written (i.e., the string values are case-sensitive)
- Numbers wrapped in quotation marks are treated as strings
- Single and double quotations can be used together. The opening and closing quotation marks must be the same. For example, we can’t start a string with a double quotation mark (“) and close it with a single quotation mark (‘).
Numbers
We can represent numbers by writing them without the quotations.
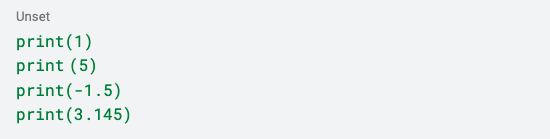
A few notes on the code:
- Numbers written without quotations are interpreted as numerical values
- A whole number (both positive and negative) is categorized as an “integer”
- A decimal number (both positive and negative) is categorized as “float”
Bool or Boolean
Human languages have the concept of correctness. For example, the sentence “elephants can fly” is incorrect or false. Similarly, computers also understand this concept. Programming languages provide a boolean data type (bool in Python) to express this concept.
In Python, the following values represent this concept:
- True: indicates correctness in Python
- False: indicates incorrectness in Python
Basic Operators in Programming
In mathematics, we can perform calculations using operators like +, -, / and so on. Programming languages also provide operators to perform calculations using values. Here’s an example of addition:

Output:

Operators are usually used within expressions to get desired results.
What is an Expression?
An “expression” in Python is any code that evaluates to a value. A single value is also an expression. Here are a few examples:
- 1 produces 1
- “Hello!” produces “Hello!”
- 5 * 2 produces 10
- num > 100 produces either True or False
An expression can contain multiple expressions within it. Let’s take a look at a more complex expression:

The above line of code has five expressions:
- 5 (produces 5)
- 2 (produces 2)
- 3 (produces 3)
- 5 + 2 (produces 7)
- (5 + 2) + 3 (produces 10)
Here’s the expression used with the print function in Python:

The computer evaluates the expression by simplifying it incrementally. Here are the intermediate steps that are hidden from us:
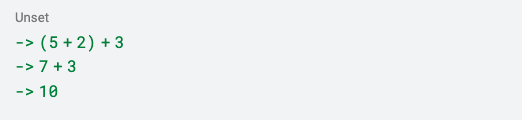
Expressions in parentheses are evaluated first.
Variables in Coding
Variables are a way to make the computer remember values. A variable is a label to which a value can be assigned.
Variables allow programmers to give meaning to values and use those values throughout the program easily. For example, the number 238,900 doesn’t mean anything but if we label it as “the distance to the Moon from the Earth” it’s easier to reference. We don’t necessarily have to remember the exact value as we can look it up using the phrase “distance to the moon from the Earth.”
Here’s how we would use a variable in Python:

We can use _ (underscore) to make big numbers easier to read in Python. Notice that we’re also using the _ to separate words in the variable name.
Output:

Variable Declaration and Assignment Walkthrough
Let’s look at how to create a variable again:

There are three parts to this line:
- cat_name is the variable name
- = is an assignment operator
- ‘Fifi’ is an expression that produces the value Fifi of type str.
So this is the general structure:

- The right side of the = operator is evaluated first
- The value produced by the expression evaluation is then assigned to the variable name or label
The line cat_name = ‘Fifi’ is usually verbalized as “declare a variable called cat_name and assign the value ‘Fifi’ to it”
Learn About Programming Variables and More at Flatiron
If you’d like to learn more about foundational concepts such as fundamental data types, programming variables, operators, and functions, check out Flatiron School’s Software Engineering Bootcamp. You’ll find info on upcoming course start dates, career paths in the field, student success stories, and an FAQ.
Disclaimer: The information in this blog is current as of March 15, 2024. Current policies, offerings, procedures, and programs may differ.