The Abstraction of Parameters
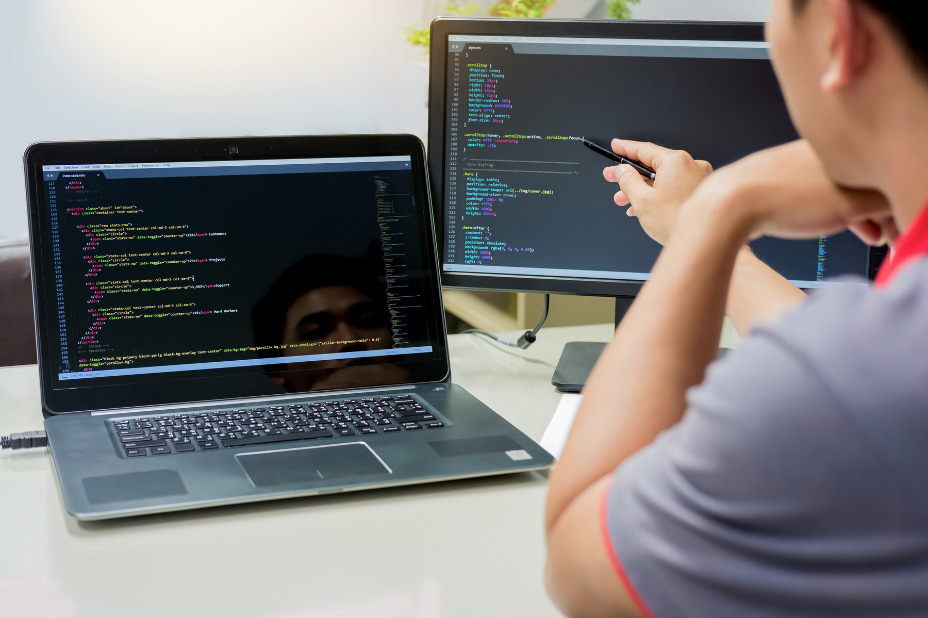
Understanding how to use the abstraction of parameters keeps your code sleek, making it easier to look for bugs later.
This article on the abstraction of parameters is part of the Content Collective series, featuring tips and expertise from Flatiron School staff members on topics ranging from program success to the job search. This series is a glimpse of the expertise you can access during your program at Flatiron School.
While programming, you may want to have the same thing happen more than once but at separate times in the code. You can copy and paste the code from the first instance, but then you have repeating code throughout your project.
While a practical solution, doing this adds many lines of code for you or others to parse through to find a bug should one occur.
To help minimize code size, stick to DRY (Don’t Repeat Yourself) code. Using functions with parameters and arguments allow you to reuse that same code over and over without adding all the lines of code each time.
What is a parameter?
A parameter is kind of like a variable that belongs to a specific function. Just like a declared function waits to be invoked or used somewhere in the code, the parameter waits to be given a value. It just sits there in the function until it is utilized and given a value.
When declaring a function you must also declare the parameter that is attached to it. To declare the parameter all you need to do is give it a name in the parentheses of the function you have declared. The syntax looks something like this in JavaScript:
function myExample(myParameter){
return myParameter
}
When giving a parameter a name you can name it anything you want to (as long as it doesn’t repeat the name of another variable or function in the same scope). However, it is helpful to others reading your code (and as a reminder to yourself) if you give the parameter a name that represents the value it is given.
For instance, if you have a function that converts Fahrenheit to Celsius, then the value you want the function to change will always be the Fahrenheit temperature. So, calling the parameter “Fahrenheit” would be ideal.
function convertToCelcius(fahrenheit){
return (fahrenheit – 32) * 5 / 9
}
In this case, the parameter called Fahrenheit will always represent the temperature to be converted to Celcius when the convertToCelcius function is invoked and given an argument.
What is an argument?
Functions exist in two states.
The first state is the declaration, or architecture of code that will happen when the function is used.
The second state is the invocation of the function. This is the signal that the code in the declaration of the function should go through its coded steps. The parameter exists in the declaration version of the function and the argument exists in the invocation of the function. The argument is the value that is given to the function and, thus, assigned to the parameter of the function.
In the previous example, the argument would be provided for that function similarly to this:
convertToCelcius(78)
This signifies to the code that the steps in the previous function example should go through its steps with the parameter (in this case called Fahrenheit) equal to 78. So, when you invoke the function like this, the code for that one time the function performs its steps is essentially the same as this:
return (78 – 32) * 5 / 9
Notice that the parameter was replaced by the argument that was given to the invocation of the function. That’s because the parameter is essentially a stand-in for the argument that is passed in. This is what makes the function dynamic and reusable with different values.
How Are Parameters and Arguments Linked?
The parameter of a function is like a container that can hold different things in it. Let’s say you have a favorite glass that you like to drink out of for every meal. On that glass, there is a label of ‘drink’. For breakfast, you have eggs and toast and your ‘drink’ glass you fill with orange juice. After breakfast, the ‘drink’ glass is empty so you can use it again for lunch (after rinsing of course). For lunch, you have a ham sandwich and fill your ‘drink’ glass with milk. Afterward, it is empty again and can be used for dinner and so on.
For each meal, the glass stays the same and always has the label ‘drink’. What changes is what the glass holds. If the meals represented a function, ‘drink’ would be a parameter in that function. Whatever it is filled with represents the argument for each meal (or the invocation of the meal function).
In Conclusion
Using parameters and arguments in your function allows you to reuse the code in the function for different purposes. This will keep your code sleek and minimize the lines you must trace back to find bugs in the code. It also shows employers that you can develop dynamic code for use in multiple places in an application.
About Joe Milius
Joe Miius is a Software Engineering Technical Coach at Flatiron School. He has previous teaching experience and has been helping Flatiron School students understand coding concepts for 2 years. He loves problem-solving and takes on each new problem or question a student presents with vigor and curiosity.
Disclaimer: The information in this blog is current as of August 9, 2023. Current policies, offerings, procedures, and programs may differ.