Software Engineering
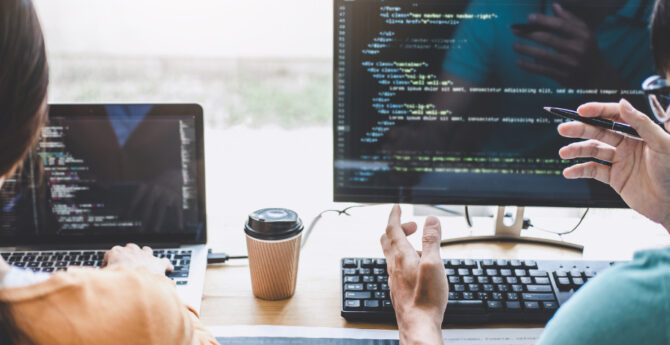
Software Engineering
11 Best Websites to Practice Coding for Beginners in 2025
Coding is not a spectator sport. To develop your skills, you must write it yourself. Here are the best websites to practice coding.
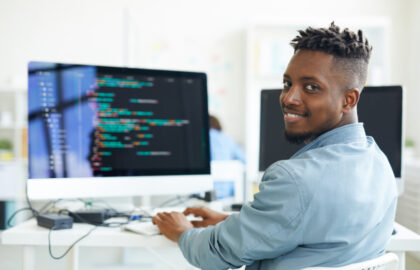
Software Engineering
7 Jobs You Can Get Knowing Python
For fledgling developers, Python is often one of the first programming languages they learn. Here are 7 jobs you could land by learning this versatile and easy-to-learn language.
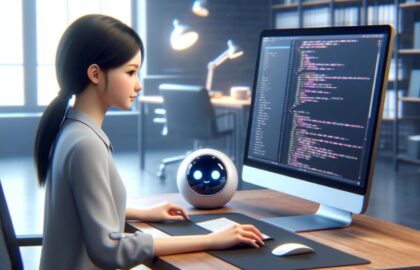
Artificial Intelligence
Software Engineering in the Age of AI
Software Engineering isn’t going anywhere soon, but proficiency in AI will quickly become essential for every Software Engineer.
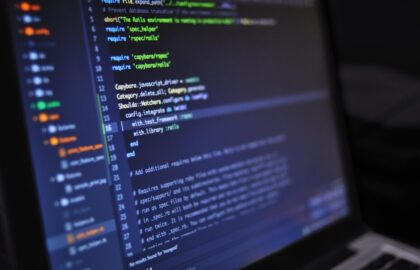
Tech Trends
The Building Blocks of Code: Understanding Programming Variables, Data Types, and Operators
Learn some of the foundational building blocks of programming languages so you can start learning to code in any language.
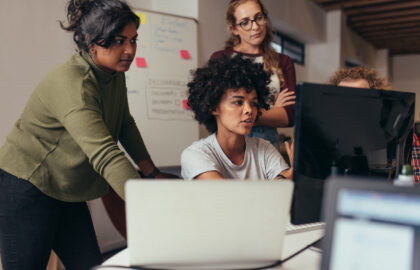
Software Engineering
Your Software Engineering Roadmap: Climbing the Career Ladder
If you’ve decided a software engineering career is for you, here’s your software engineering roadmap and guide to climbing the career ladder.
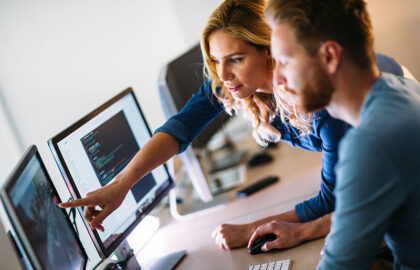
Software Engineering
Is JavaScript Hard to Learn? Everything to Know About the Most Popular Programming Language
While JavaScript isn’t necessarily as difficult to learn as you may have heard, it may take some time to get up to speed. Read this article for an overview of what you need to learn.
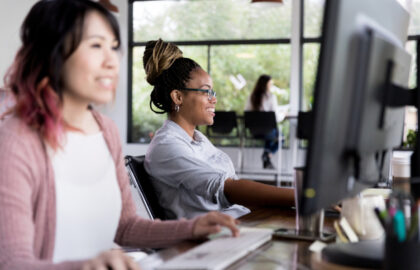
Women In Tech
Women In Tech: 4 Grad’s Stories | Women’s History Month
In celebration of Women’s History Month, here are the stories of four recent female Flatiron School grads making waves in the tech industry.
Browse by Category
- All Categories
- Admissions
- Alumni Stories
- Announcements
- Artificial Intelligence
- Career Advice
- Cybersecurity Engineering
- Data Science
- Denver Campus
- Diversity In Tech
- Enterprise
- Flatiron School
- How To
- NYC Campus
- Online Campus
- Partnerships
- Software Engineering
- Staff / Coach Features
- Tech Trends
- UX / UI Product Design
- Women In Tech