The Power of Variables: Storing and Using Information
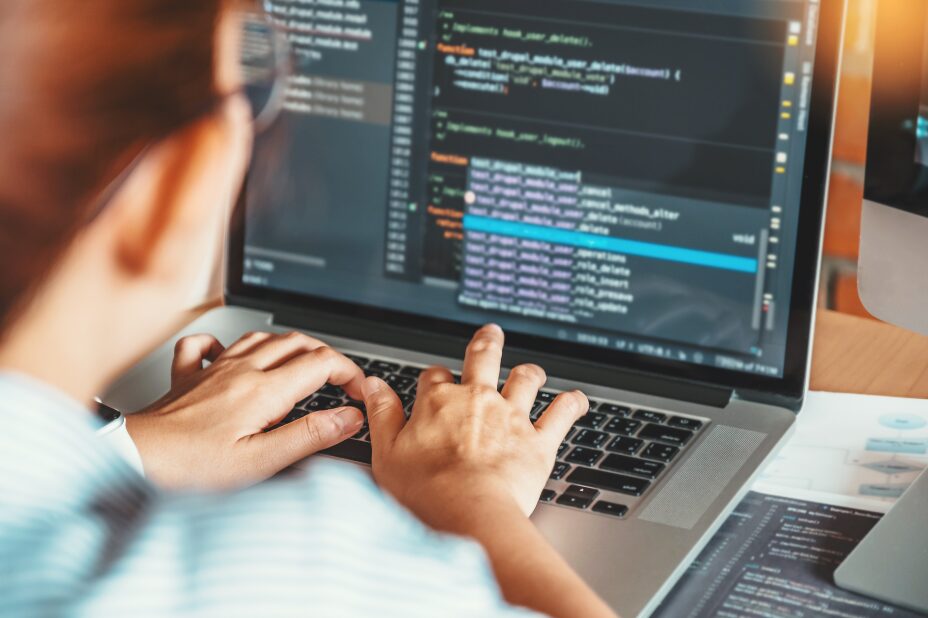
Variables are one of the most important concepts in programming. Read on to learn how to think about them intuitively.
One of the most important foundational building concepts in programming is variables. Variables are a way to make a computer remember values. A variable is a label to which a value can be assigned.
Variables allow programmers to give meaning to values and use those values throughout the program easily. For example, the number 238900 doesn’t mean anything but if we label it as “the distance between the Moon and the Earth” it’s easier to reference. We don’t have to remember the exact value as we can look it up using the phrase “distance between the Moon and the Earth.”
Here’s how we would use a variable in Python:

Output:

We can use “_”
(underscore) to make big numbers easier to read in Python. Notice that we’re also using the “_”
to separate words in the variable name.
Variable Naming Conventions
It is important to follow consistent guidelines when naming variables. The projects you work on will likely have their own guidelines. (Check out Google’s Python style guide as an example.)
The following are common Python variable naming conventions used in the industry:
- Variable names can only contain letters, numbers, and underscores
(_)
. - They can only start with a letter or an underscore (
greeting1
and_greeting1
are valid but1greeting
is not). - Variables are case-sensitive (
greeting
andGreeting
are separate variables). - Multiple words must be separated using
_
(my_birthday
,number_of_people
). This convention is called snake_case. - Variable names must be meaningful (
max_speed
instead ofm
).
Visualizing Computer Memory
Whenever we ask the computer to represent a string, number, or boolean it creates the representation in its memory. You can think of computer memory as a giant container that can store information for the duration of a program’s execution.
At the start of program execution, the computer’s memory is empty. We can visualize it like this:
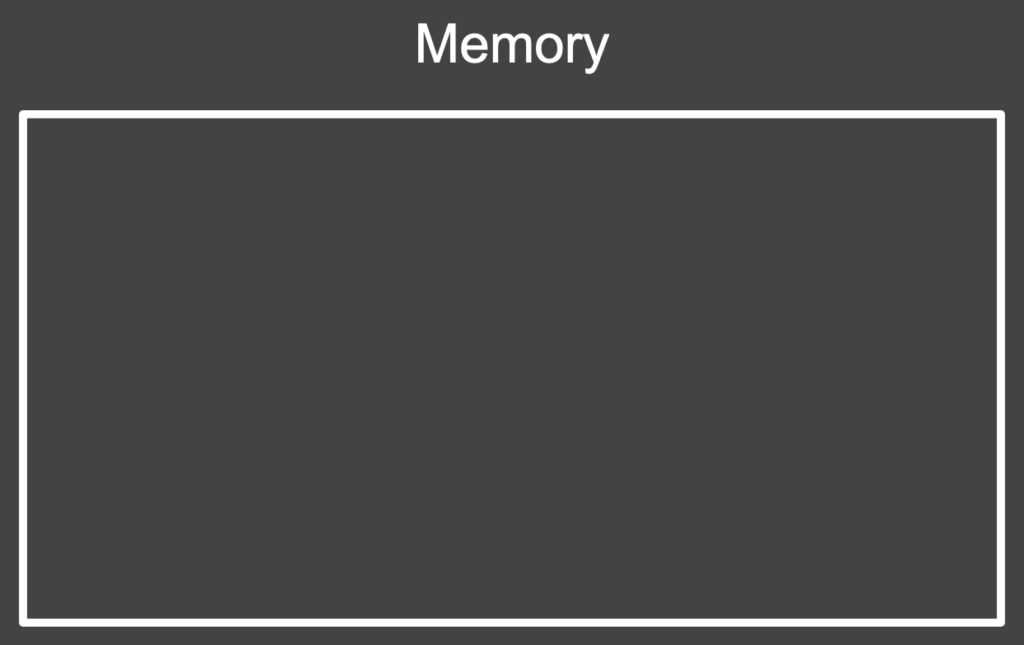
Now let’s run some code and visualize how the memory will change during execution:

For each of the values, a representation will be created in the memory during execution.
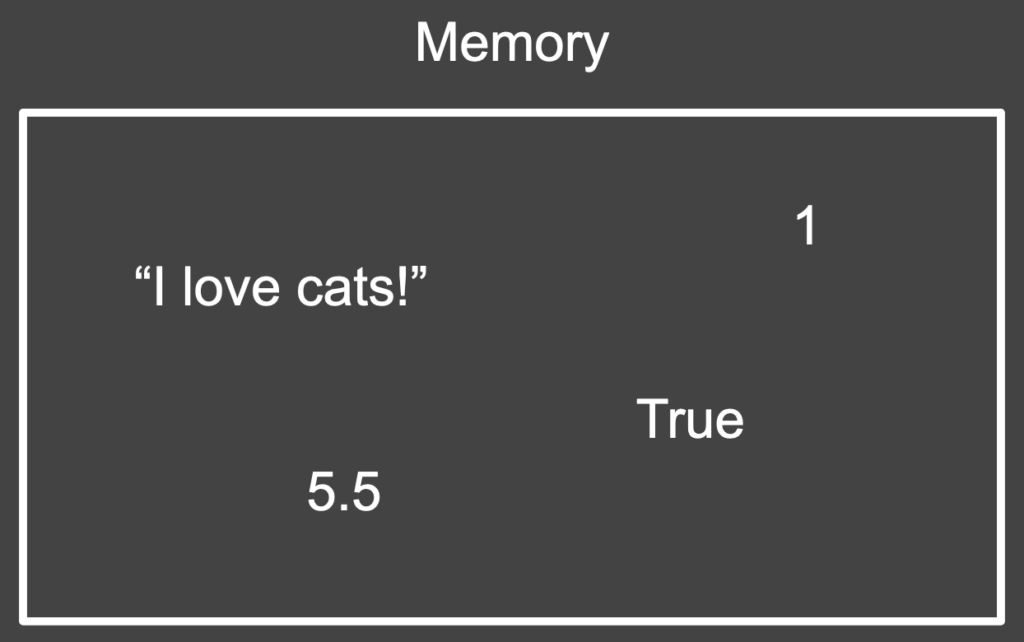
Once the program completes execution, the memory will be empty again.
Note that if we had two print(1)
statements, there would still be only a single representation of the integer 1
. This is the case for strings and booleans too.
Variable Declaration and Assignment
Let’s look at how to create a variable again:

There are three parts to this line:
cat_name
is the variable name.=
is an assignment operator.'Fifi'
is an expression that produces the valueFifi
of typestr
.
So this is the general structure:

- The right side of the
=
operator is evaluated first. - The value produced by the expression evaluation is then assigned to the variable name or label.
The line cat_name = 'Fifi'
is usually verbalized as “declare a variable called cat_name and assign the value ‘Fifi’ to it.
Visualizing declaration and assignment
We are going to visualize the following line:

First, the computer evaluates the expression which in this case produces the string 'Fifi'
and stores it in the memory.
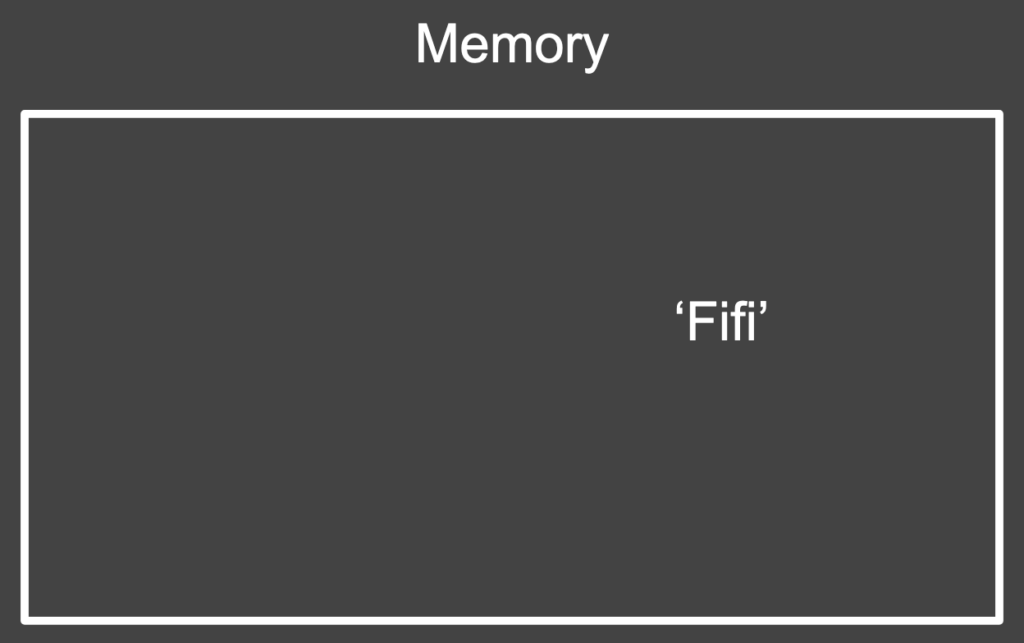
The computer would then declare the variable with the given name. Notice that the string is in ''
while the variable name is not.
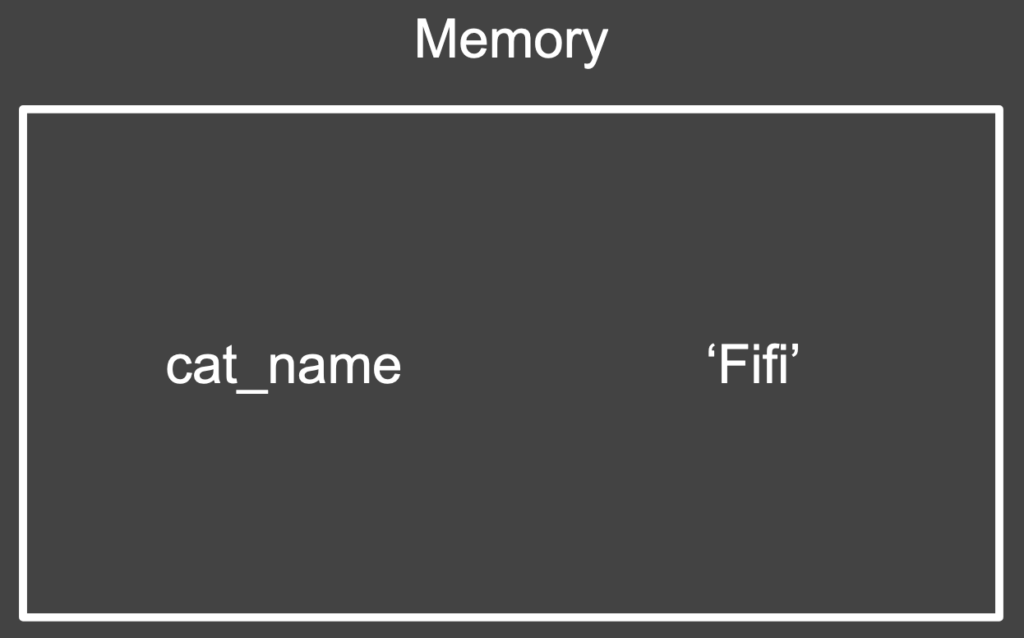
Finally, the computer makes the variable name point to the value (i.e., it assigns the value to the variable label).
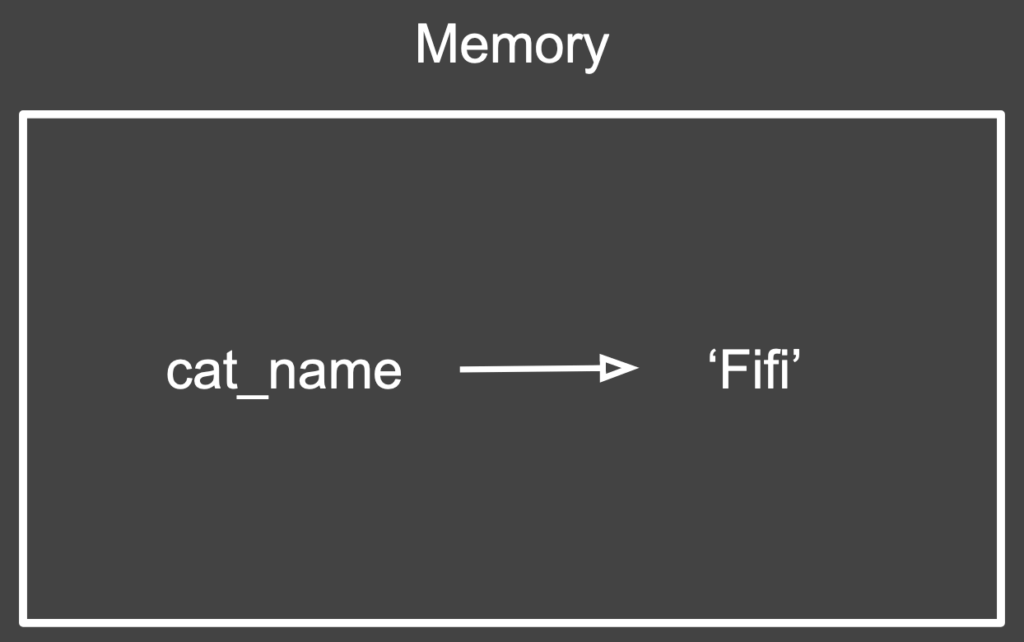
Variable Access
We’ve seen how a computer declares variables and assigns values to them. Now we will look at what happens when the computer accesses a variable.
Here’s the code we will be working with:

When a computer sees a variable label it checks its memory for the label. If the label exists, the value that the label points to is used by the computer.
We can visualize this by checking the memory diagram and following the arrow from the variable label (cat_name
) to the value (’Fifi’
).
Variable Reassignment
Variable values can be changed during the execution of the program. For example:

The first line will result in the following memory state:
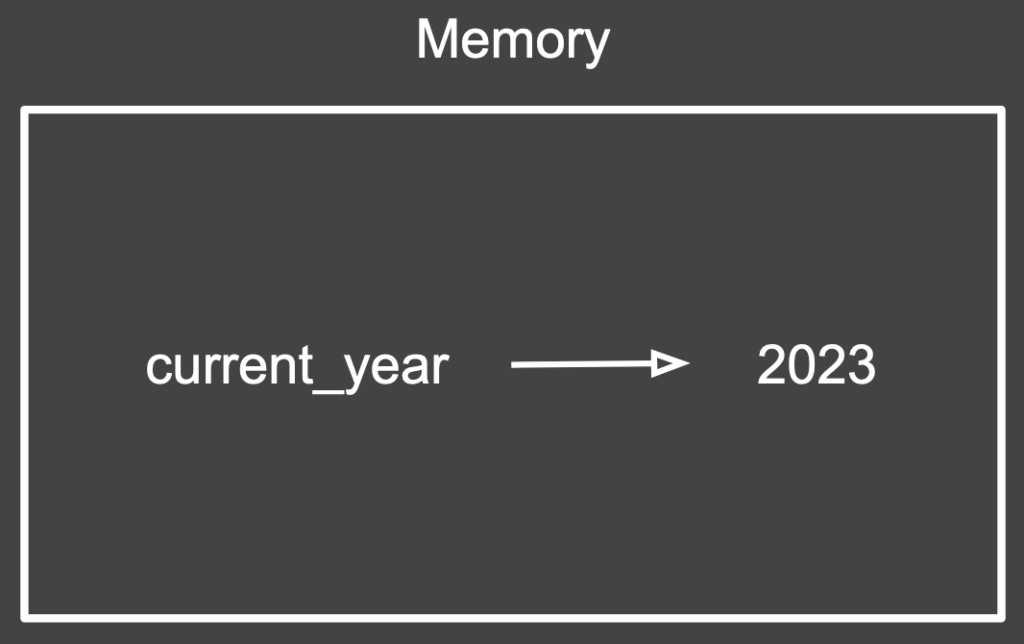
On the second line, the computer will look up the current_year
label in its memory and display the value 2023
.
With the third line, a few things happen:
- The computer creates the representation of
2023
in its memory (remember the right-hand side of the = operator is evaluated first).
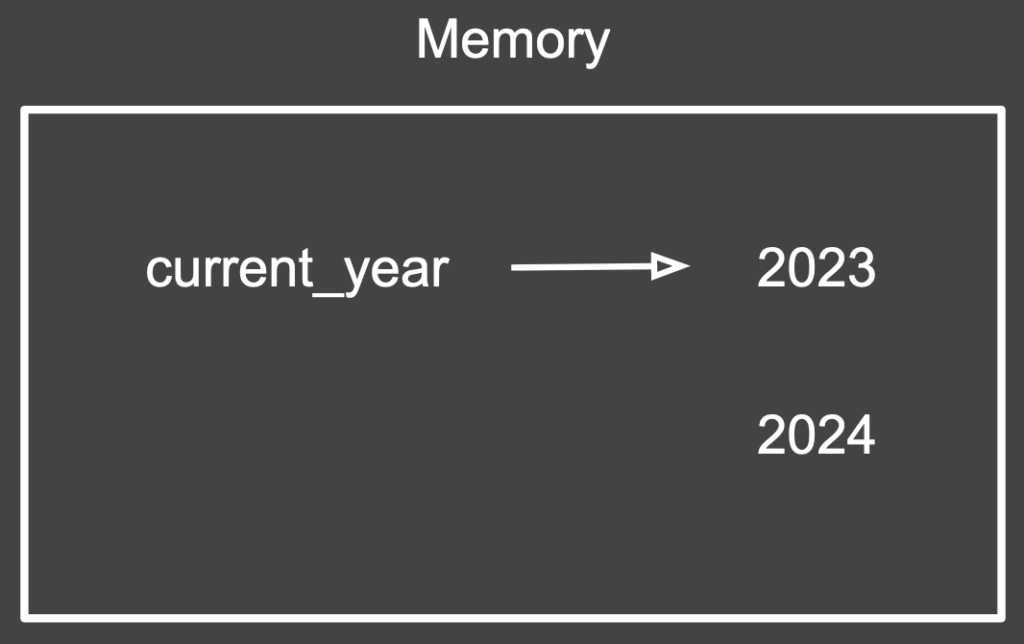
- The
current_year
label arrow is pointed to the2024
representation.
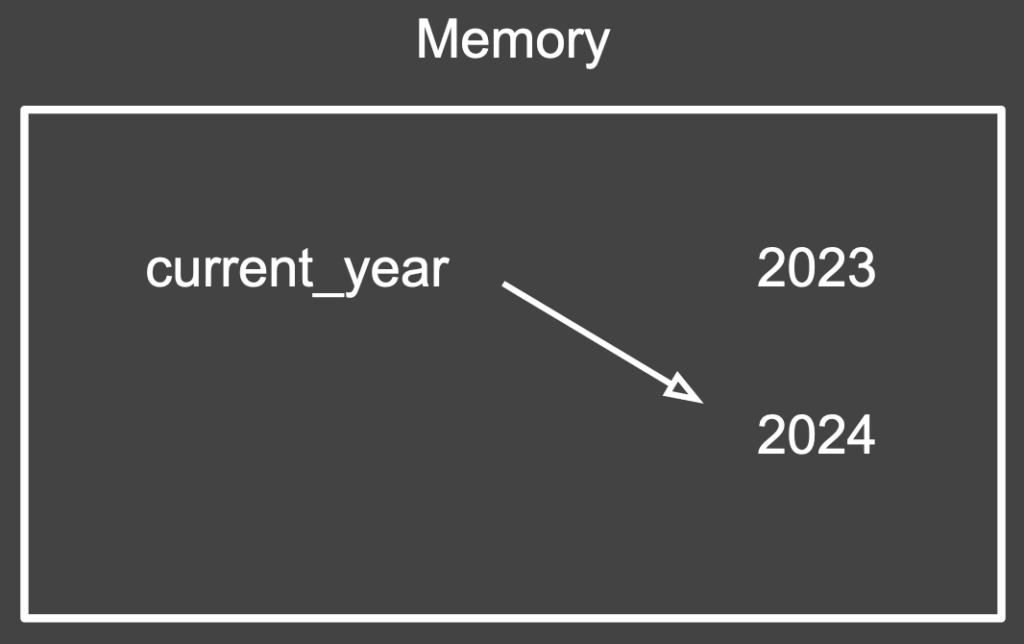
- Since no variables are pointing to the
2023
value anymore, it gets removed from memory.
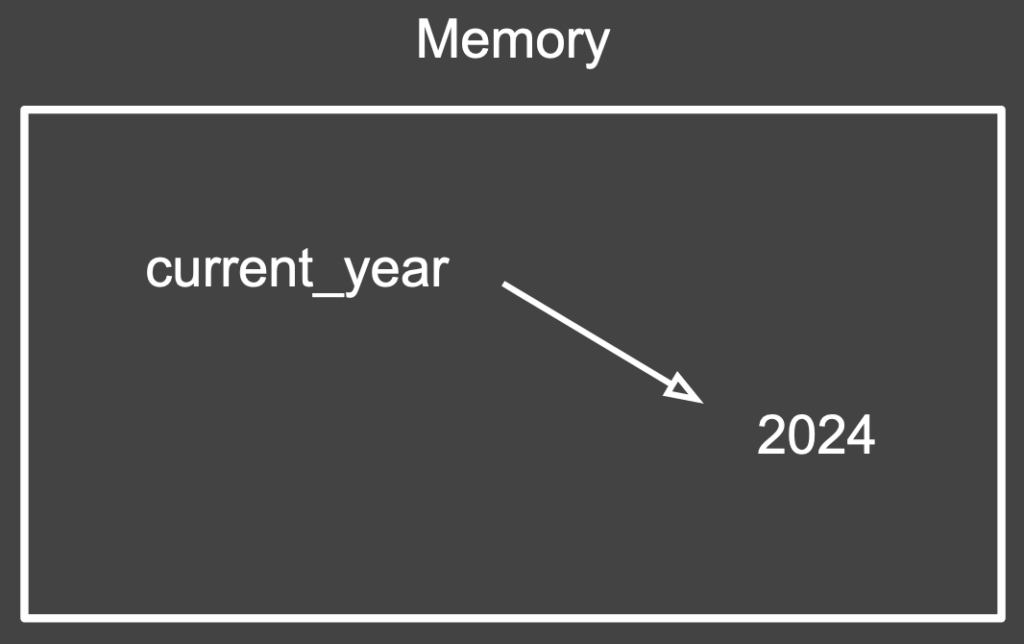
On the 4th and final line, the computer checks its memory for the current_label
again but this time it’s pointing to a new value (2024
).
As a reminder, this mental model works for strings and booleans too!
Hopefully you now have an understanding of how to visualize variable declaration and assignment. These visualizations can also be used for reference type values such as arrays and objects. Whenever you’re using variables, make sure to follow a consistent guideline and identify what value a variable is referencing. With these foundations, you will be able to effectively use variables in any programming language.
Make a Software Engineering Career Change at Flatiron
Our Software Engineering Bootcamp offers hands-on training, expert technical coaching, and real-world projects designed to launch your career in tech. Don’t miss this opportunity to join a vibrant community of developers and unlock limitless possibilities. Apply now and fast-track your path to success in the dynamic field of software engineering.
Disclaimer: The information in this blog is current as of April 12, 2024. Current policies, offerings, procedures, and programs may differ.